TLFC
provides all the tools you need to write end to end typesafe AWS lambda functions. It comes with schema validation at runtime on the client and the lambda, a local dev environment and a simple CLI to build and deploy your functions into AWS.
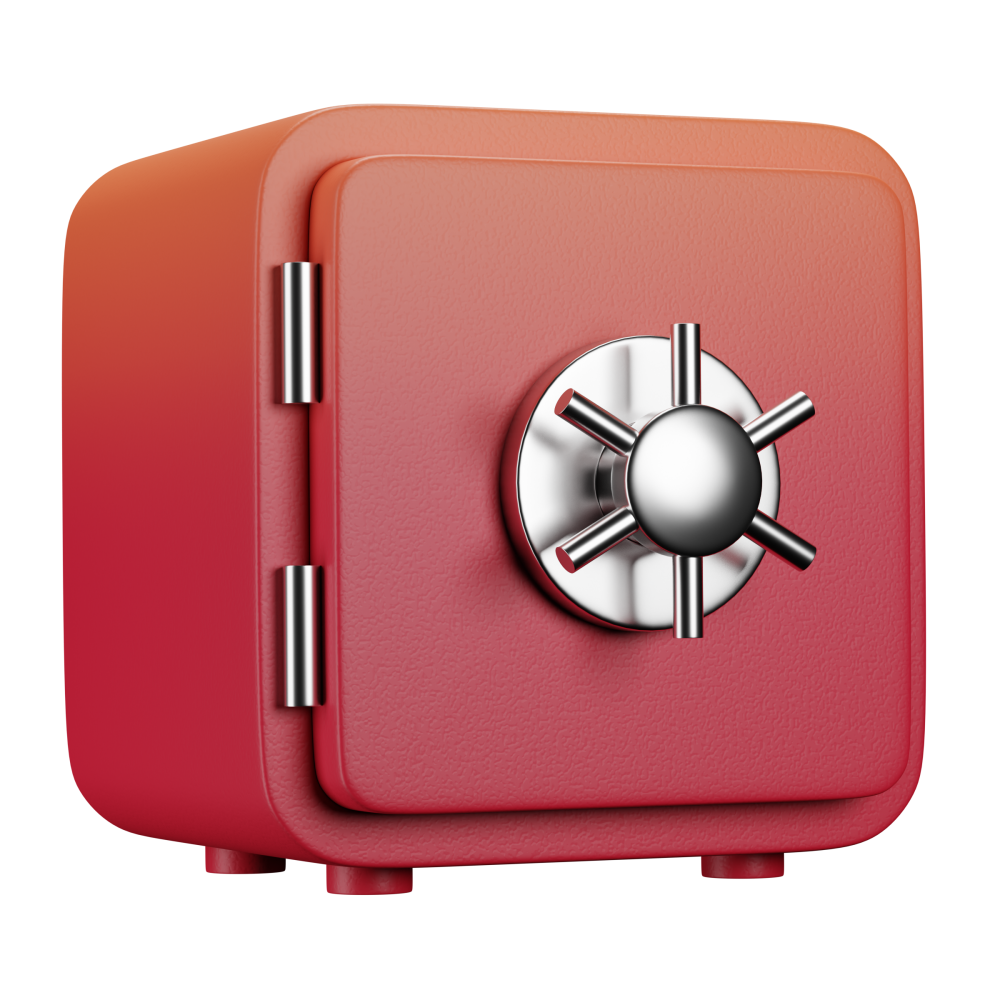
Typesafe
TLFC is typesafe out of the box. It ensures a strict interface between the client and the lambda. By relying on zod's schema validation TLFC ensures typesafe network calls without any extra development work.
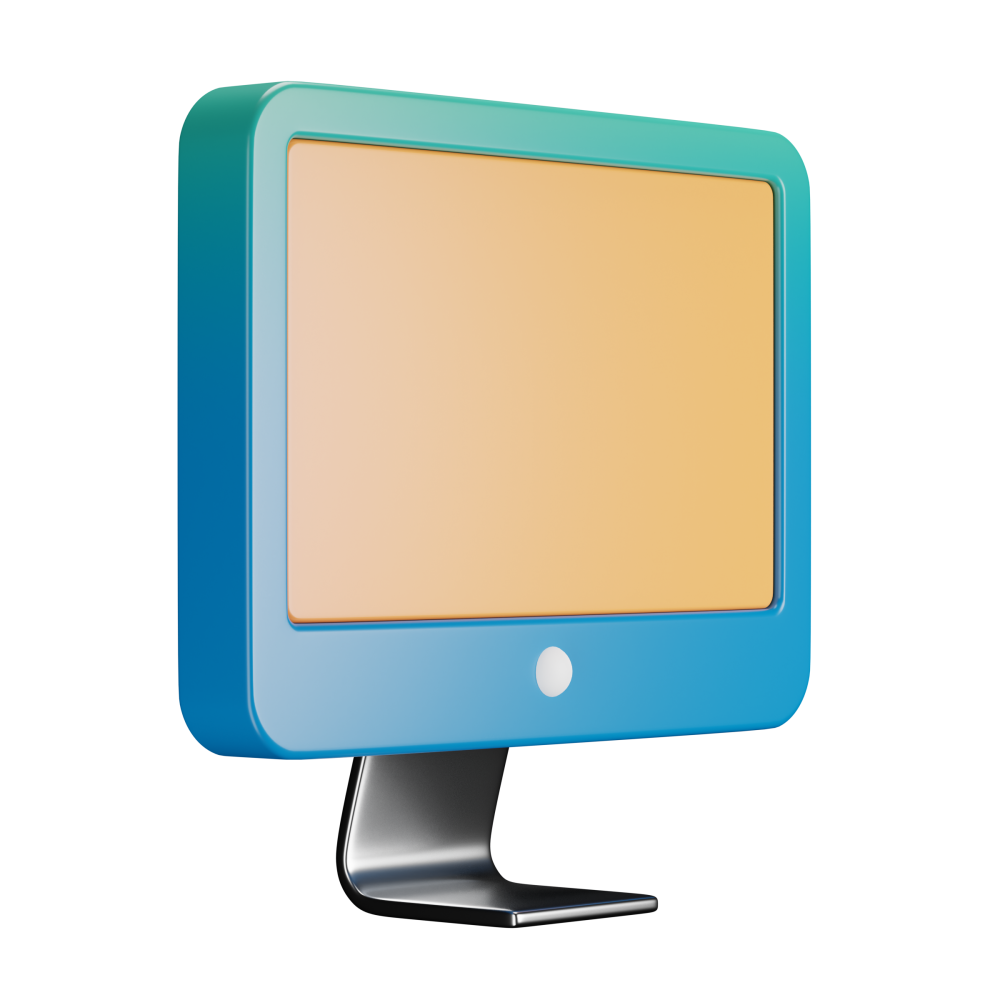
Development experience
TLFC provides a great development experience. It has nearly no configuration and provides an easy to use API. By creating an abstraction over the network layer implementing a lambda fetch feels like calling a function.
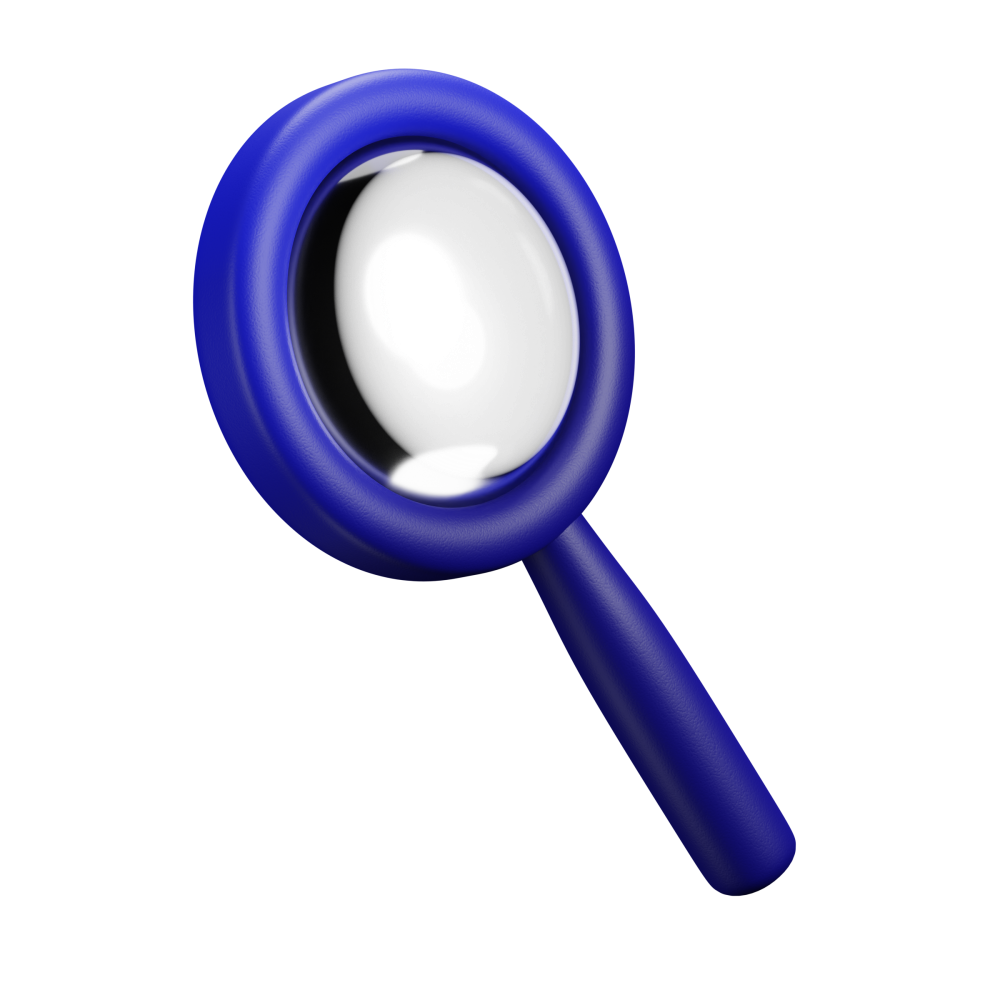
Payload validation
To ensure absolute safety TLFC not only performs checks on compile-time. It also validates the request payloads in the lambda and response payloads in the clients at run-time.
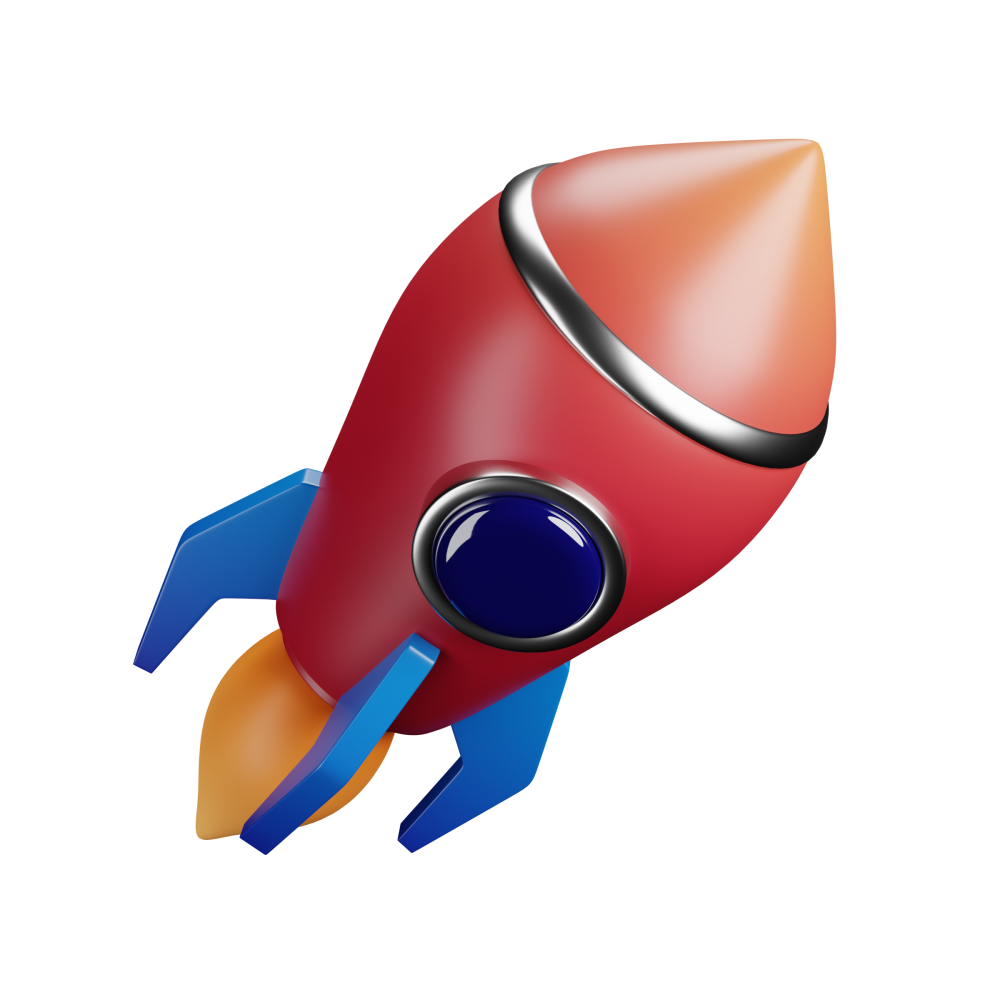
Batteries included
TLFC brings all utilities to run a local dev environment, bundle the lambdas and even deploy to AWS. It provides a CLI, a programmatic interface and plugins to integrate into you frontend toolkit.
Get started
import { z } from "zod";
import { createLambda } from "@tlfc/server";
export default createLambda(
{
requestSchema: z.object({ name: z.string() }),
responseSchema: z.object({ msg: z.string() }),
functionName: "greetingHandler",
},
async (event) => {
return {
msg: `Hello ${event.name}`,
};
}
);
🏗️ Create a simple lambda function:
In order to ship your first lambda you need to make use of the createLambda utility from the @tlfc/server package. The object that is created by this function call needs to be the default export of the file, so the dev server and deployment can pick up the lambda definition.
Use zod to define the schemas of your lambda's request and response format. The schemas are used to validate the requests that the lambda should process and the response that the client receives.
You also need to define a functionName for your lambda. This will be used to identify the endpoint where the lambda can be reached.
import greetingLambda from "./greeting-lambda";
const response = await greetingLambda.call({
name: "test",
});
console.log(response.msg);
📞 Call the Lambda from you client:
To call the lambda function just import the function export from your lambda file and use the call utility.